Algorithms: Insertion sort
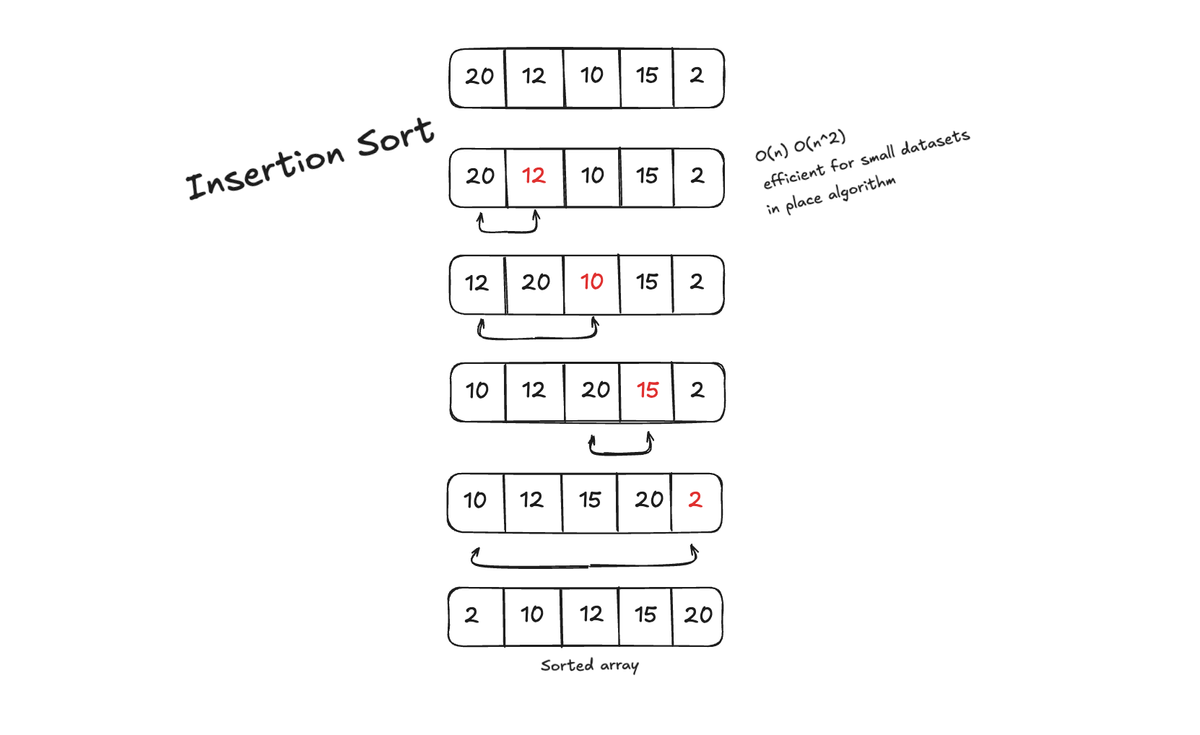
Next algorithm I want to learn more about is the Insertion sort algorithm. This algorithm works like we humans sort playing cards by hand.
This algorithm works by building a sorted portion of the array one element at a time. For each step in an array we do the following.
- Pick the next element from the unsorted portion
- Compare it with elements in the sorted portion
- We insert it into the correct position within the sorted portion.
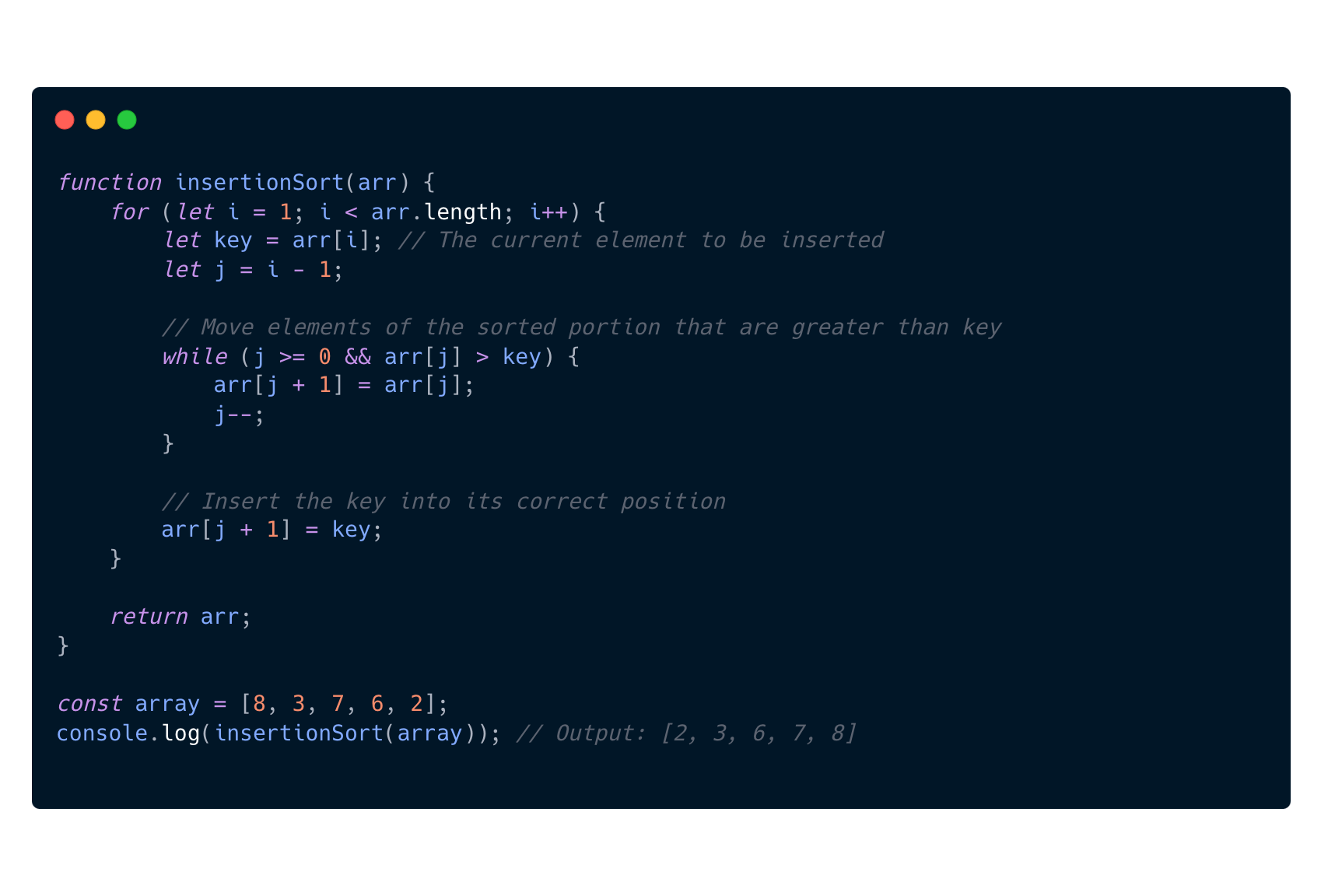
In this example we can have the mind visualisation as follows.
- We start by picking up cards one by one
- We place each card in the correct position relative to the others we have already sorted.
Key takeaways of Insertion sort:
- It is easy to understand and implement
- It is efficient for small datasets
- In-place algorithm and don't need extra memory to sort arrays.
- It can be inefficient for larger datasets
- We have repeated comparisons. For each element, comparisons are repeated even if unnecessary in larger arrays.
So now when we understand the basics of Insertion sort and the complexity that comes with it we must practice this together.
Here is some basic -> Expert exercises for Insertion Sort
Comparison with other sorting algorithms
Algorithm | Time Complexity (Best) | Time Complexity (Worst) | Space Complexity | Stable? |
---|---|---|---|---|
Selection | No | |||
Bubble | (optimized) | Yes | ||
Insertion | Yes | |||
Merge | Yes | |||
Quick | No |
Algorithms: Quick Sort
So last time we learnt more about divide and conquer algorithms and Merge Sort. Lets continue down that road and look on an eficient sorting algorithm called Quick Sort. This algorithm at glance is simliar to Merge Sort but it works a little bit different. If you have not read
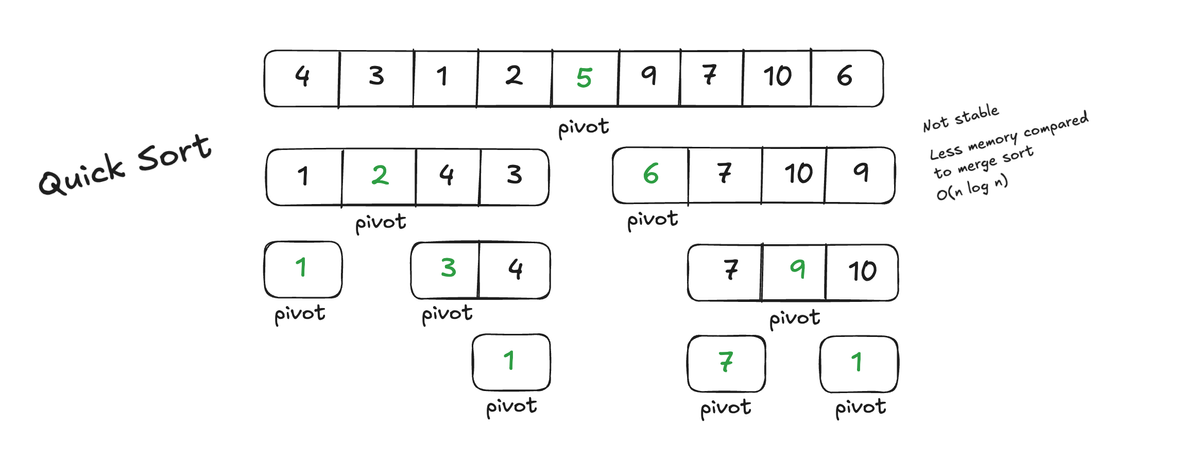
Algorithms: Merge Sort
Now we have goon through Bubble sort and Selection sort and as we have learned it is not efficient algorithms by any means. Selection sort is even unstable. So lets turn our perspective to one of the most efficient sorting algorithms. Let me introduce the Merge sort algorithm. Merge sort
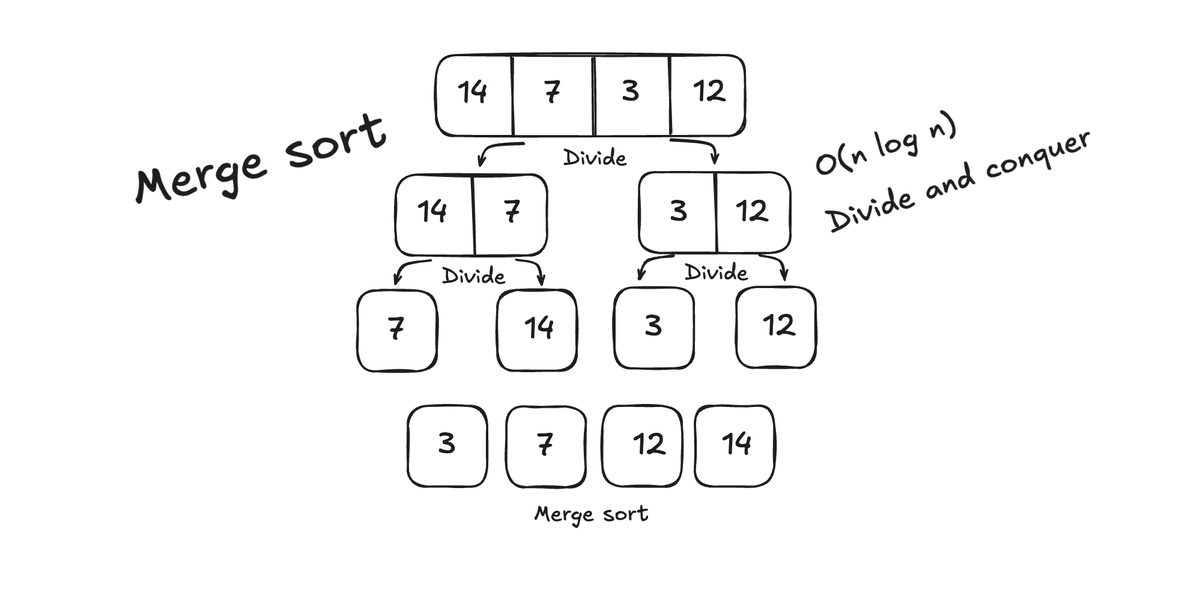